December 7, 2014 | Share | Comment
I'm a huge consumer of the radio station Radio 24/7, mainly through their iPhone app. Unfortunately it sometimes acts funny; e.g. if I unplug the headphones from my iPhone usually the app using the audio-output will stop playing -- this is not the case with this app, which sometimes can lead to some interesting situations when the app suddenly starts playing when I least expect it.
Thus I have decided to try to reverse-engineer the app to make it better. This is an unusual path to take, but the app has not been updated in ages, so now I'm just being proactive.
The process
I imagine there are a few steps to go through before the app is ready for deployment:
- Investigate the possible end-points to use as streaming-source and program information.
- Outline a simple layout and decide Swift or Objective-C should be used.
- Development!
I will create a separate post for each step.
March 1, 2014 | Share | Comment
I'm working on a minor project to find the daily average price on (currently only) iPhones on a major danish trading site called DBA.dk. Currently I managed to get access to their internal API by "reverse engineering" how they communicate with it through their iPhone App.
Backend
I'm building the backend (scraper and REST server) using Scala and will probably be using some of the libraries available by Spray.io. I have just started exploring Scala and is learning a little every time I'm using it - but progress is slow as I'm currently hung up on various other projects (thesis). The book Programming in Scala by Martin Odersky (the designer of Scala) et al. is by the way highly recommendable! The backend code is available on Github.
Frontend
For quite a long time I have thought about trying out AngularJS but haven't found a suitable project, but for this I think it fits perfectly. I'm planning on decoupling frontend and backend completely, and let the frontend communicate with the backend through AJAX calls, by doing this the actual website can be hosted on e.g. Github free static site host.
As I by no means am a designer I will of course just be using Bootstrap as design.
Faking an API
Now to the reason for this post: I'm trying to find a service where I can make fake endpoints available to test against when developing the frontend. I ran across this simple project on Github: JSON Server, essentially just a (very) simple server serving static JSON content. It can be used as a Node module or just as a CLI tool directly, e.g.:
$ cat db.json
{
"posts": [
{ "id": 1, "body": "foo" }
]
}
$ json-server --file db.json
$ curl -i http://localhost:3000/posts/1
For learning AngularJS I think it's a great tool as no effort need to be put into the backend before the actual requirements to it has been covered by developing the frontend.
February 8, 2014 | Share | Comment
In this blog post I will be focusing on configuring MongoDB to be used on a server with Ubuntu as OS. The MongoDB manual has some nice pages on how to secure MongoDB on different OS, including Ubuntu.
Firstly MongoDB should be installed (preferable with MongoDB downloads repository) as described here. After that we need to create an admin user, initiate mongo
:
$ mongo
> use admin
> db.addUser({user:"admin", pwd:"PASSWORD",roles:["userAdminAnyDatabase"]})
To gain access to the server from outside iptables should be configured to allow access to and from port 27017, the default MongoDB server port.
Now all we need to do is to make sure the auth
-flag is set on the mongod
instance currently running. Uncomment the #auth = true
line in the file /etc/mongodb.conf
and restart the MongoDB service: sudo service mongodb restart
. Now you should only be able to access the server with credentials when the host is not localhost.
The admin user created is not very useful, we need to create some databases with users:
$ mongo
> use admin
> db.auth("admin", "PASSWORD")
> use test-database
> db.addUser({user:"test-user", pwd:"PASSWORD",roles:["readWrite","dbAdmin"]})
Essentially we just created the database test-database
with the user test-user
which has permission to read, write data and create new collections (see the user privileges for MongoDB).
February 7, 2014 | Share | Comment
For this I will be using Homebrew (a very useful package manager for Mac OS), if you don't know this you should head over to their homepage and get it installed right now, or just enter the following command:
ruby -e "$(curl -fsSL https://raw.github.com/Homebrew/homebrew/go/install)"
Removing an existing MongoDB installation
A year ago I installed MongoDB and used it in a small node.js project and haven't used it since, in the meantime I have (of course) lost the passwords for the local development database, so the first step is to completely remove the existing installation of MongoDB.
First I removed MongoDB through Homebrew:
Then I ensured that the mongod
binary was shutdown through the Activity Monitor. After that I removed the possible left-overs (data-files, etc.) located by inspecting the Brew-formula for MongoDB. These files were located in /usr/local/var/mongodb
, so this folder was completely removed:
rm -r /usr/local/var/mongodb
Installing MongoDB
After that I just installed MongoDB again:
brew update
brew install mongodb
launchctl unload ~/Library/LaunchAgents/homebrew.mxcl.mongodb.plist
launchctl load ~/Library/LaunchAgents/homebrew.mxcl.mongodb.plist
And verified that it worked:
Giving the output:
MongoDB shell version: 2.4.9
connecting to: test
>
For easy listing of databases, collections and data the the MongoHub GUI is strongly recommended.
The next blog post will be about creating users with appropriate permissions.
January 19, 2014 | Share | Comment
A while ago I started using this domain as my personal web page (not in the current form), at that time I started using GitHub Pages to host my simple index.html
page.
When I added this blog to the domain I realised that I need something more powerful than just simple html-files. Firstly I knew that I still wanted to use GitHub Pages to host the domain, this meant I needed to find some kind of static website generator. Secondly I knew that I wanted to use the Markdown format to generate blog posts and thirdly I wanted a very simple layout.
Finding a layout
The research began and I found several options, mostly based on Jekyll, a static website generator. I found Jekyll Bootstrap which comes with a few features out of the box (comments, tags, categories, etc.) but with the default Twitter Bootstrap theme. Then I found Jekyll Themes which has a bunch of different themes to use with Jekyll. But I couldn't find a very simple, elegant layout.
At last I came across Poole, a simple foundation for Jekyll. It has two themes of which I chose Hyde.
Setting it all up
I downloaded the master-branch from the GitHub page, extracted the content into a folder and issued:
This will fire up a server on http://localhost:4000 and watch the files in the directory for changes. After having customised the sidebar and added Disqus comments to blog posts it was time to write the first post.
I'm using the Markdown editor Mou for Mac where the markdown is previewed live constantly:
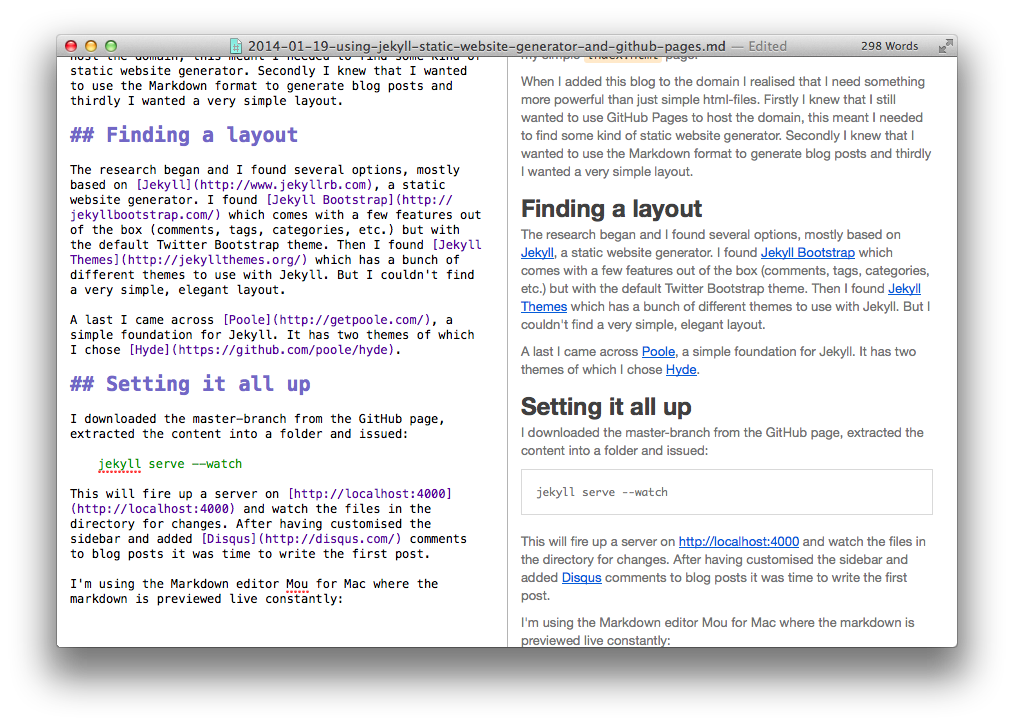
Alternatively the markdown can be viewed directly in the browser by updating the localhost path where the specific post is placed - given that the Jekyll command above has been issued.
Using GitHub pages
Finally to host everything on GitHub the repository USERNAME.github.io
has to be created and everything that should be accessible must be pushed to this repo. GitHub Pages support Jekyll out of the box, thus when a repository with the Jekyll directory structure is pushed, the static pages are generated instantly when accessed through the url http://USERNAME.github.io
.
To use a custom domain the DNS settings should be updated as described here: https://help.github.com/articles/setting-up-a-custom-domain-with-pages.
That's about it. A simple version-controlled, free hosted blog engine is set up. Blog posts can even by submitted directly through the GitHub repository web interface, as GitHub supports creation of new files via the web interface.
Go to https://github.com/jboysen/jboysen.github.io to see the details of the config-file and directory structure of this site.